Python break facilitates the early exit from a loop. Control structure provides certain loops such as Python for loop, while loop, which helps in executing a block of code as many times as required. As a programmer, you can choose any looping structure which serves the purpose. On many occasions, you don’t want the loop to continue executing till the end but it should exit upon meeting certain criteria. In this article, you will learn about the Python break, continue and pass.
Table of contents
- Significance of Python break, pass and continue
- Example of the “break” in various Python loops
- Example of the “pass” in various Python loops
- Example of the “continue” in various Python loops
- Conclusion
Significance of Python break, pass and continue
In this section, you will learn about break, pass and continue in Python. Let’s first understand the terminology along with the flow diagram and later you will see the implementation in the code.
Pass
The pass statement is a null operation in Python, which means, nothing happens when it executes, but is required to avoid generating errors. Python pass keyword is used to create the code skeleton without popping up any syntactical error during code compilation. At many places, you want to design a python control structure or function but do not want them to execute during compilation. In such a scenario, you can use the “pass” keyword. You can find the example of “pass” in subsequent sections.

Break
Python break is used to get an early exit from the loop (be it for loop or while loop). Consider a scenario, where you want to execute a loop from beginning till the end but at the same time, you also want the loop to terminate upon meeting certain criteria in between the execution. You will see an example of a break with different loops in the subsequent section.
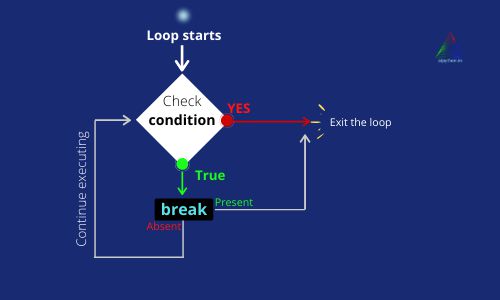
Continue
In Python, continue keyword is used to skip the current execution and control of the loop pints at the beginning of the loop. Consider a scenario where you want to skip the current execution upon meeting a certain condition then you can use continue keyword. You will see an example of a continue with different loops in the subsequent section.
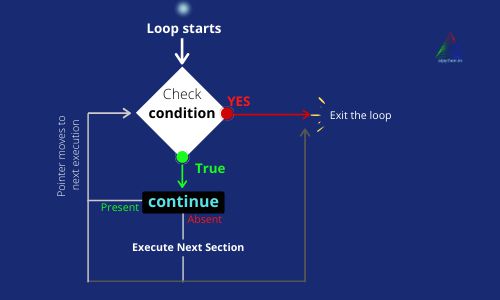
Example of the “break” in various Python loops
In this section, you will learn the usage of “break” in all the control structure format.
Python break in for loop
for i in range(10):
print ("Inside loop and value is: ", i)
if i == 4:
break
print ("Now outside loop after break")
Output:
Inside loop and value is: 0
Inside loop and value is: 1
Inside loop and value is: 2
Inside loop and value is: 3
Inside loop and value is: 4
Now outside loop after break
Python break while loop
x = 5
while x < 15:
print ("Number is less than 15")
if (x == 7):
print ("Number is equal to 7")
break
x += 1
print ("Outside while loop")
Output:
Number is less than 15
Number is less than 15
Number is less than 15
Number is equal to 7
Outside while loop
Example of the “pass” in various Python loops
In this section, you will learn the usage of “pass” in all the control structure as well as function.
Pass in for loop
s = "aipython"
for char in s:
pass
Output: >>
Pass in while loop
i = 0
while i < 5:
i+= 1
if i == 2:
pass
print (f"Current value is {i}")
print ("Outside the loop")
This keeps on running infinitely and you have to manually terminate the execution by raising a keyboard interrupt like CTRL+C.
Pass in the if-else statement
s = "aipython"
i = 0
if i < len(s):
pass
print ("Outside if statement")
Output:
Outside if statement
Pass in Python user define function
The pass can also be placed inside the body of a function.
Why a programmer do this?
A programmer needs to start coding even in the absence of all the requirement document and in these situations, he/she knows about the presence of function but not the action that function is supposed to do. In this scenario, A pass function is very helpful.
def func_name ( ):
pass
Example of the “continue” in various Python loops
In this section, you will learn the usage of “continue” in a various control structure format.
Continue in Python for loop
s = "aipython"
for i in s:
if i == 'p':
print ("Letter p is found and skipping ...")
continue
else:
print ("Current letter is :" + i)
print ("Outide the loop")
Output:
Current letter is :a
Current letter is :i
Letter p is found and skipping …
Current letter is :y
Current letter is :t
Current letter is :h
Current letter is 😮
Current letter is :n
Outide the loop
Continue in Python while loop
s = "aipython"
i = 0
while i < len(s):
if s[i] == 'p':
print ("Letter p is found and skipping ...")
i += 1
continue
else:
print ("Current letter is :" + s[i])
i += 1
print ("Outide the loop")
Output:
Current letter is :a
Current letter is :i
Letter p is found and skipping …
Current letter is :y
Current letter is :t
Current letter is :h
Current letter is 😮
Current letter is :n
Outide the loop
Conclusion
After reading this tutorial you must have gained enough knowledge to use Python break, pass and continue in the program. You have learned how to use them in the various control structures of Python.
Now, I hope you can utilize the knowledge to build your code more robust and clean.